Understanding Variable Scope in Software Management Systems: Coding Standards
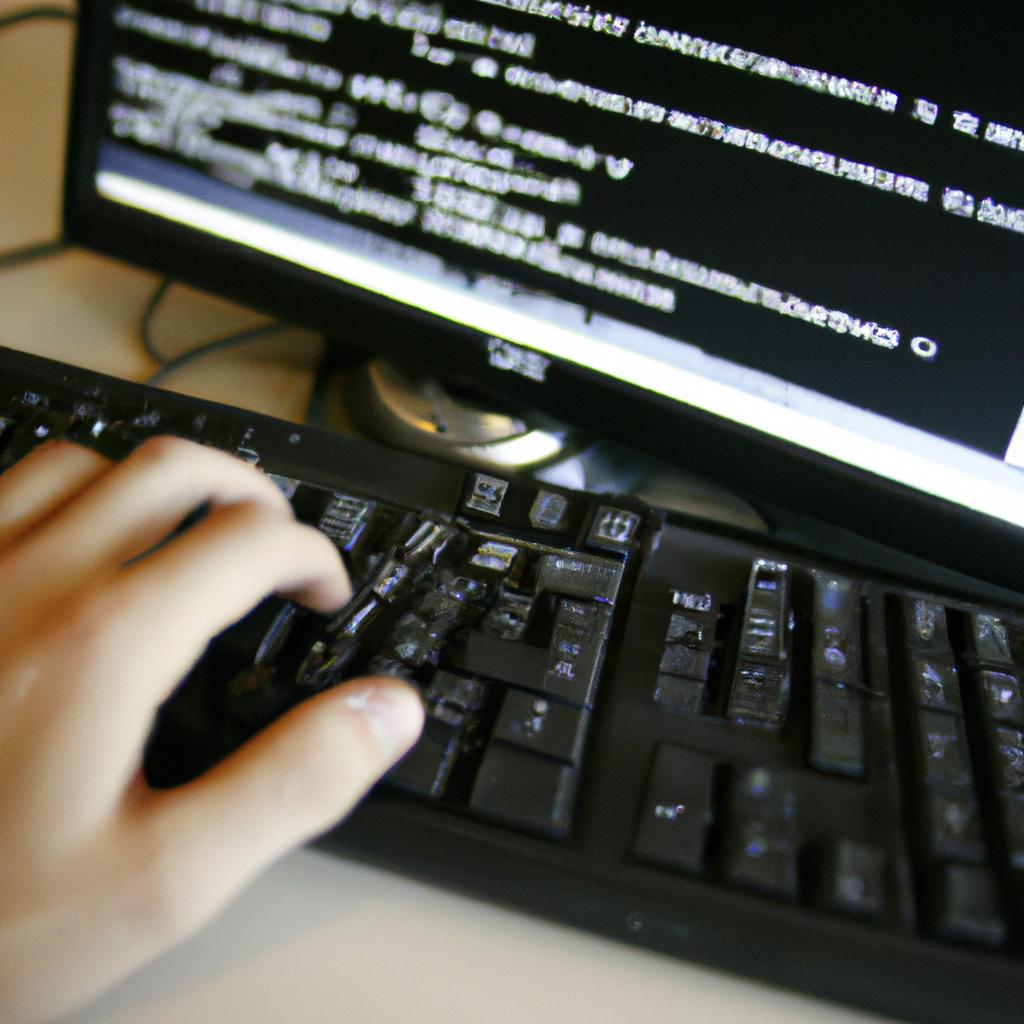
In the world of software development, understanding variable scope is crucial for maintaining code quality and consistency. Variable scope refers to the range in which a specific variable can be accessed within a program. When implementing coding standards, it is important to establish guidelines regarding variable scope to ensure that programming practices are consistent across all projects.
For example, consider a hypothetical scenario where an organization has multiple developers working on separate components of a larger system. Without clear guidelines on variable scope, each developer may implement their own approach leading to confusion and errors when integrating different modules together. By establishing coding standards that include rules for variable scope, such as limiting global variables or using descriptive names for local variables, these potential issues can be avoided.
This article will explore the importance of understanding variable scope in software management systems and provide guidance on how best to establish effective coding standards around this concept. We will delve into various scenarios where proper management of variable scope can lead to more efficient and reliable code and discuss common mistakes made by programmers in relation to this topic. Ultimately, we hope readers will gain greater knowledge about this fundamental aspect of software development and feel empowered to apply it effectively in their own projects.
Importance of Proper Variable Scope in Software Management Systems
The importance of proper variable scope in software management systems cannot be overstated. Variable scope refers to the part of a program where a particular variable can be accessed and manipulated. Improper usage or absence of variable scope could lead to bugs, errors, and security vulnerabilities that may compromise the entire system.
For instance, consider an e-commerce website that stores users’ personal information such as names, addresses, and payment details. If the developers fail to implement appropriate variable scopes for these variables, an attacker who gains access to the system could potentially steal sensitive data from all users registered on the platform without being detected.
To emphasize further why proper variable scope is crucial in software development, here are some key points:
- Security: As mentioned earlier, lack of proper variable scoping poses significant risks to system security.
- Maintainability: When codebases grow larger over time with multiple contributors working on them simultaneously, it becomes increasingly challenging to maintain them efficiently. Properly scoped variables make it easier for developers to understand how different parts of a program interact with each other.
- Efficiency: Inefficient use of resources like CPU cycles and memory could lead to slower application performance. By limiting the scope of variables within functions or blocks where they are needed reduces unnecessary resource consumption by releasing memory when no longer required.
- Readability: Code readability makes debugging more manageable because it allows faster identification of logical flaws in programs.
In addition to understanding why proper variable scoping is essential in software development let us examine some common types of variable scopes used across different programming languages:
Scope Type | Description | Lifetime |
---|---|---|
Global | Variables declared outside any function or block have global scope; thus accessible throughout the program. | Until program terminates |
Local | A local variable is one that has been defined inside a specific function or block; its accessibility limited only within that particular block or function. | Until the execution of a specific function |
Block | In some programming languages, blocks like if-else statements and loops can declare their own variables; these are called block-scoped variables. They exist only within the confines of that block. | Until execution of the code inside the block is complete |
In conclusion, proper variable scope in software management systems plays an integral role in ensuring system security, maintainability, efficiency, and readability. Different programming languages implement various types of variable scopes to achieve these objectives effectively. The next section will delve into more detail about each type of variable scope used across different programming languages without further ado.
Types of Variable Scope in Programming Languages
After understanding the significance of proper variable scope in software management systems, it is essential to explore various types of variable scopes utilized in programming languages. Let’s take an example of a hypothetical program that calculates the total price for purchasing items from an online store.
There are mainly two types of variable scopes: global and local. Global variables have no restrictions on their usage within a program, whereas local variables only exist within specific functions or blocks of code. It is crucial to consider which type of variable scope suits your program requirements before starting coding.
The use of global variables may seem convenient at first glance; however, they can cause issues such as naming conflicts and unintended changes by other parts of the codebase. On the contrary, using local variables provides better control over data flow within the program and avoids unexpected behaviors.
Here are some pros and cons associated with both types:
-
Global Variables
- Pros:
- Easy access throughout the entire application
- Facilitates communication between modules
- Cons:
- Naming conflicts
- Unintentional modifications by unrelated code
- Pros:
-
Local Variables
- Pros:
- More controlled data flow
- Avoids unpredictable behavior
- Cons:
- Limited accessibility outside its block
- Pros:
Moreover, there are different ways to declare local variables depending on where they reside within functions or loops. For instance, declaring them inside a loop means every iteration creates new copies rather than modifying existing ones.
Variable Scope | Declaration Location | Lifetime |
---|---|---|
Local | Inside function | Until function ends |
Block | Inside curly braces | Until block ends |
In conclusion, choosing appropriate variable scopes is necessary for ensuring efficient programs’ development without any errors or bugs. The next section will discuss the benefits of using local variable scope specifically.
Benefits of Using Local Variable Scope
After learning about the types of variable scope in programming languages, let’s dive deeper into why it is important to use local variable scope.
Consider a scenario where a software developer is working on a large project and decides to declare all variables as global for ease of access. While this may seem like an efficient solution at first, it can lead to several issues down the line. For instance, if two functions are using the same global variable, one function could unintentionally change its value which would affect the second function.
To avoid such problems, developers should follow coding standards that enforce the use of local variable scope. Here are some reasons why:
- Reduced risk of bugs: When variables have limited visibility within specific blocks or functions, there is less likelihood of conflicts between different parts of the codebase.
- Improved readability: Local variables clearly define their purpose and lifespan within a particular block or function making it easier for other developers to read and understand the code.
- Enhanced maintainability: Using local scope makes it easier to make changes later on without affecting other parts of the codebase unnecessarily.
- Increased security: By limiting access to sensitive data through carefully managed scopes (e.g., private versus public), you reduce potential vulnerabilities from malicious attacks.
Table: Benefits of Using Local Variable Scope
Benefit | Description |
---|---|
Reduced Risk Of Bugs | Fewer chances for conflicts between different parts of codebase |
Improved Readability | Easier understanding by other developers |
Enhanced Maintainability | Changes can be made without unnecessary impact |
Increased Security | Limiting access reduces vulnerability |
It is worth noting that while using local variable scope brings many benefits, it requires extra attention from developers when designing software. This means taking time during development stages to plan out how each piece fits together with regards to inheritance chains or dependency injection strategies so as not only ensure optimal performance but also prevent errors that can occur from variable naming conventions.
Common Mistakes in Variable Scope Management
After understanding the benefits of using local variable scope, it is important to be aware of common mistakes in variable scope management. One example that illustrates how variable scope can go wrong involves a software developer who was working on an e-commerce website. The developer created a global variable to store customer information for convenience when processing transactions. However, this decision led to security issues as any code within the project could access sensitive data such as credit card numbers and addresses.
To prevent similar problems from occurring, software teams should educate themselves about common mistakes in variable scope management. Here are some key points to keep in mind:
- Avoid creating global variables unless absolutely necessary
- Always declare variables with the smallest possible scope
- Be cautious when reusing variables across different functions or modules
- Use descriptive names for variables to avoid potential naming conflicts
Furthermore, it is helpful to understand the differences between static and dynamic scoping. Static scoping refers to how a programming language determines which value a variable has based on its location within nested blocks of code. Dynamic scoping, on the other hand, determines which value a variable has based on the current state of execution at runtime. Understanding these concepts will help developers make more informed decisions about how they manage their code’s memory allocation.
To fully grasp the importance of proper variable scope management, consider the following table showcasing common errors along with their potential impacts:
Error | Impact | Example |
---|---|---|
Global Variable Usage | Security Breaches | Storing sensitive user data globally |
Overwriting Variables | Unexpected Behavior | Reusing counter variables in nested loops |
Inconsistent Naming Conventions | Confusion and Errors | Using ‘i’ for both index and iterator variables |
Unused Variables | Unnecessary Memory Consumption | Declaring but not utilizing large arrays |
By being mindful of these pitfalls and incorporating best practices into your workflow, you can improve your team’s coding standards and ensure a more efficient and secure software development process.
Next, let’s explore the best practices for implementing variable scope in your project, which can help mitigate these common errors and improve overall code quality.
Best Practices for Implementing Variable Scope
After understanding the common mistakes in variable scope management, it is crucial to implement best practices for smooth software development. In this section, we will discuss some of the most effective methods for implementing variable scope.
One of the best ways to ensure proper variable scope implementation is by following coding standards that specify guidelines and rules for code structure. These standards may vary from one organization to another but often include conventions such as naming conventions, indentation, and comments. Adhering to these standards can help developers maintain consistency while writing code, making debugging easier and more efficient.
Another important practice when working with variables is initializing them before use. Failing to initialize a variable could lead to unpredictable behavior or even crashes at runtime. Thus, it’s essential always to set default values explicitly or provide initial values during declaration.
It’s also worth noting that overusing global variables should be avoided since they can cause conflicts between different parts of the program. Instead, consider encapsulating all data inside functions or classes where possible. This way, you limit access only within those areas where they’re necessary instead of exposing them globally.
Implementing unit tests can significantly help detect issues earlier on in the software development lifecycle . Unit testing ensures that each part of your application works correctly individually before being integrated into larger components. It reduces errors caused by interdependent modules and makes debugging much easier when an issue arises.
Finally, documenting your code throughout its lifecycle helps prevent confusion when others work with it later down the line . Clear documentation enables other team members to understand how various components interact with each other and what their purpose is quickly.
To summarize, adhering to coding standards for consistent style formatting, initializing variables before using them, avoiding global variables whenever possible through encapsulation techniques like functions or classes; implementing unit tests early on in development cycles; maintaining clear documentation are essential steps towards successful variable scoping implementations.
Pros | Cons |
---|---|
Improved readability and maintainability of code. | Time-consuming to set up initially, can feel like extra effort for developers. |
Reduced likelihood of bugs and errors in the software development cycle. | Can be overlooked or forgotten by team members who are not as familiar with coding standards. |
Encourages better communication between team members working on the same project. | Inflexibility – may limit functionality if adhering strictly to guidelines |
Provides a foundation for teamwork when multiple programmers work together on the same project. | Can increase time spent reviewing/checking that every line aligns with coding standard rules. |
Tools and Techniques for Debugging Variable Scope Issues will be discussed next in this article
Tools and Techniques for Debugging Variable Scope Issues
After implementing best practices for variable scope, it is important to utilize tools and techniques to debug any potential issues that may arise. For example, consider a hypothetical scenario where a software team has implemented proper variable scoping but encounters an error in their code.
To begin debugging the issue, developers can use logging statements to track the flow of data throughout the program. This allows them to identify where variables are being defined and used, as well as any unexpected changes in their values. Additionally, using breakpoints in an integrated development environment (IDE) can help pinpoint specific lines of code where errors occur.
Despite these efforts, some variable scope issues may still persist. In such cases, teams should consider utilizing static analysis tools which scan code for potential bugs and offer suggestions for improvement. These tools can provide insights into overlooked areas of code or suggest alternative approaches to variable scoping that may improve overall efficiency.
However, while automated tools can be helpful in detecting errors, they cannot replace human judgement entirely. Developers must remain vigilant and continue testing their code manually to catch any issues that automated systems might overlook.
It is also essential for coding standards to be established within a software organization regarding variable scope best practices. A clear set of guidelines ensures consistency across all projects and minimizes the risk of avoidable mistakes occurring due to misunderstandings or misinterpretations.
In summary, implementing best practices for variable scope is critical for maintaining efficient and reliable software management systems. While there are various methods available for debugging potential issues that may arise, it is crucial not to solely rely on automated technologies without manual oversight from experienced developers who understand how works in practice. Establishing coding standards will further ensure consistent application of these practices across all projects undertaken by a software organization.
- Potential benefits:
- Improved performance
- Reduced risk of errors
- Greater ease-of-use
- Increased scalability
Pros | Cons |
---|---|
Improved code readability | Initial time investment required for establishing coding standards |
Better maintainability | May require additional training and resources to implement effectively |
Reduced risk of bugs and errors | Can be difficult to enforce consistently across a large organization |
Increases efficiency in development process |