Coding Standards: Software Management System Best Practices
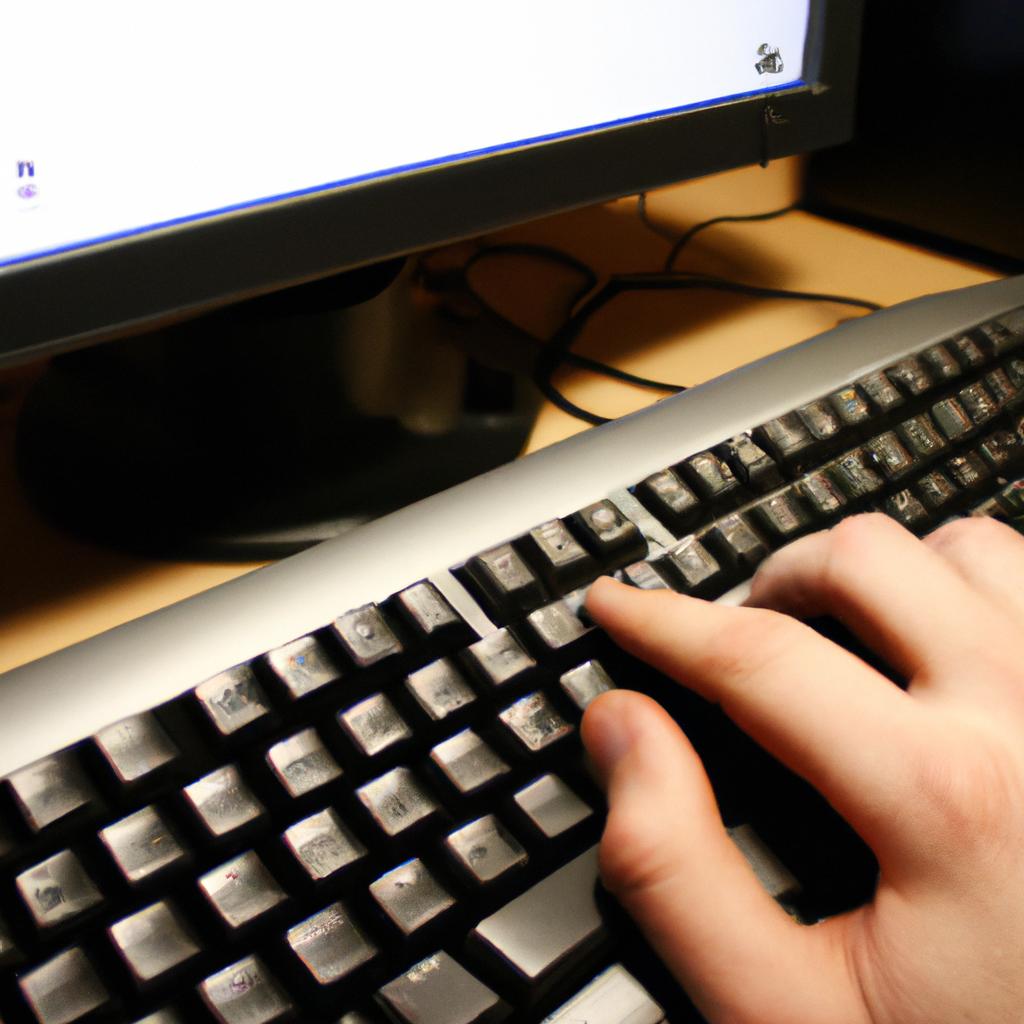
In today’s fast-paced world, software development has become an indispensable part of businesses. As companies strive to bring their products and services online, the need for software management systems (SMSs) has increased exponentially. SMSs are designed to automate processes and workflows that were once manual, time-consuming, error-prone tasks. However, as with any system implementation, there are risks associated with SMS adoption.
One such risk is the lack of coding standards within a company’s software development team. Coding standards refer to a set of guidelines that outline how code should be written and formatted in order to make it easier to read, maintain and debug. In short, coding standards ensure consistency across all levels of programming within an organization. For instance, consider a hypothetical scenario where Company A’s software developers have no standardized approach for writing code. One developer might use tabs while another uses spaces; one writes long functions whereas another keeps them short and concise. This inconsistent approach leads to confusion among developers and makes debugging difficult when issues arise down the line – costing Company A both time and money in lost productivity.
Consistent naming of variables and functions
Consistent naming of variables and functions is an essential aspect of coding standards that ensures readability, maintainability, and scalability of software management systems. For instance, consider a hypothetical case where two developers worked on a project called “OpenAI Response.” One developer named their variable as “,” while the other used “oar_kw” instead. The inconsistency in naming makes it challenging to understand code for anyone else who reviews or maintains the codebase.
To ensure consistent naming conventions throughout the project, following are some best practices:
- Choose descriptive names: Variables and function names should accurately describe what they represent. Avoid using abbreviations or acronyms unless widely accepted by the industry.
- Follow a standardized format: Establishing standard naming patterns such as camelCase or snake_case can make code easier to read and maintain.
- Use meaningful prefixes or suffixes: Prefixes like “is_” for Boolean values or “_id” for foreign keys help differentiate between similar-looking variables and improve clarity.
- Avoid reserved words:. Use unique names that don’t clash with built-in language keywords to prevent errors.
Adhering to these guidelines not only helps reduce confusion but also enhances collaboration among team members working on complex projects involving multiple modules.
In addition to consistency in naming, proper indentation is another crucial factor affecting code quality. Using appropriate whitespace characters at defined intervals improves code readability by creating structure within blocks of code.{| class=”wikitable”
! Language !! Style !! Example |
---|
Python |
print("Hello World!")
|-
| C# || Tabs || void Foo()
{
\tConsole.WriteLine(“Hello World!”);
} |
---|
Java |
System.out.println("Hello World!");
} |}
The above table illustrates different programming languages and their preferred indentation styles. It’s important to note that consistency is crucial not just within a single file but also throughout the entire codebase.
In conclusion, consistent naming of variables and functions plays an integral role in developing high-quality software management systems. Standardizing conventions can prevent confusion and enhance collaboration among team members. Furthermore, using proper code indentation for readability helps create structure and improves maintainability. The subsequent section will discuss how to use appropriate whitespace characters for indentation without sacrificing efficiency or aesthetics.
Using proper code indentation for readability
Consistent naming of variables and functions is crucial to the readability and maintainability of software. The next best practice in coding standards is using proper code indentation for readability. Proper indentation allows developers to easily identify logical blocks of code, making it easier to read and modify.
For example, consider a hypothetical scenario where two developers are working on the same project but have different styles of indentation. Developer A uses four spaces while developer B uses tabs. This inconsistency leads to confusion among team members as they try to navigate through the codebase. By adhering to consistent indentation practices, this problem can be avoided.
Using proper code indentation also makes it easier to detect errors in the code. For instance, if there is an issue with the nesting of conditional statements or loops, improper indentation could make it difficult for developers to spot such errors until runtime. However, when a standard format is used across all lines of code, it becomes much easier to notice any inconsistencies that may indicate potential issues.
In addition to consistency and error detection benefits, proper code indentation enhances collaboration among team members by facilitating communication between them. When everyone follows the same style guide, it’s much simpler for people who didn’t write a particular section of code to understand how it works without having to ask questions about each line individually.
To encourage adherence to proper code indentation practices within your development team:
- Educate new hires on your company’s specific guidelines during orientation.
- Use automated tools like linters or integrated development environments (IDEs) that can automatically apply formatting rules.
- Conduct regular peer reviews where developers assess each other’s work for compliance with agreed-upon coding standards.
- Reward good behavior by publicly acknowledging those who consistently adhere to these practices.
Adopting consistent coding standards that include proper use of indentation will lead not only better quality software but also a more productive and collaborative team environment.
Pros | Cons |
---|---|
Eases readability of code | Time-consuming to enforce |
Makes it easier to detect errors in the code | Some developers may find it restrictive |
Enhances collaboration among team members | New hires may struggle to adapt initially |
Leads to better quality software | May require additional training for existing employees |
In conclusion, adopting proper indentation practices is essential for improving the maintainability and readability of your code. By adhering to a consistent style guide, you can enhance communication between team members, reduce confusion, and make it easier to identify potential issues before they become major problems. In our next section about “Adding meaningful comments to clarify code functionality,” we’ll discuss how another best practice can further improve the effectiveness and efficiency of software management systems.
Adding meaningful comments to clarify code functionality
As software development projects become more complex, it is critical to ensure that code readability is maintained. In addition to proper indentation, adding meaningful comments can significantly enhance the clarity and comprehension of written code.
For example, consider a case where a developer writes a function that calculates the distance between two points on a map. The function may seem straightforward at first glance; however, without any explanatory comments, someone else reviewing the code for maintenance or upgrades might struggle with understanding its purpose and functionality.
To clarify code functionality further, here are some best practices for writing meaningful comments:
- Use clear language: Comments should be written in simple, concise language so that everyone who reads them can understand their meaning.
- Explain why: It’s not enough to explain what the code does; you also need to describe why it does it. Understanding how something works is essential when making changes down the line.
- Avoid redundancy: Do not repeat what the code already communicates clearly. Instead, provide additional context or explanation about areas of confusion or complexity.
- Keep comments up-to-date: As your code evolves over time, update your comments accordingly.
The importance of clear communication extends far beyond just commenting. A lack of cohesion within coding standards across an organization often leads to misunderstandings and wasted time spent cleaning up errors caused by inconsistency in style choices.
Consider this table comparing three different styles for naming variables:
Variable Name | Camel Case | Snake_case | PascalCase |
---|---|---|---|
Example Value 1 | exampleValue1 | example_value_1 | ExampleValue1 |
Example Value 2 | exampleValue2 | example_value_2 | ExampleValue2 |
Example Value 3 | exampleValue3 | example_value_3 | ExampleValue3 |
While each approach has its merits depending on organizational preference and specific project requirements, choosing one standard helps create consistency and save valuable time during both initial development and subsequent maintenance efforts.
In conclusion, following best practices for commenting code can improve readability and comprehension, leading to more efficient development. Clear communication across an organization in coding standards is crucial when it comes to reducing errors caused by inconsistency.
Implementing code reusability to reduce redundancy is another critical aspect of software development that we will explore in the next section.
Implementing code reusability to reduce redundancy
After implementing meaningful comments, the next step towards achieving software management system best practices is to adopt code reusability. Code reuse involves writing reusable pieces of code that can be used in multiple places throughout the software development process. The concept behind this practice is to reduce redundancy and improve overall efficiency.
Consider a hypothetical example where a company develops two different projects requiring similar functionalities. A developer could either write the same piece of code twice or extract it into a separate module for reuse across both projects. The latter approach saves time and effort while reducing errors associated with redundant coding efforts.
To implement code reusability, developers need to follow some best practices:
- Modular design: Divide your program into small modules that are self-contained and independent of other modules.
- Standardize interfaces: Standardizing interfaces between modules ensures easy integration and reduces coupling between them.
- Build libraries: Build libraries containing commonly used functions that can be easily integrated into any project.
- Use open-source libraries: Open-source libraries provide ready-made solutions for common problems, saving developers significant amounts of time and effort.
In addition to these best practices, another essential aspect of code reusability is version control. Version control systems allow you to keep track of changes made over time and revert back to earlier versions if necessary.
Library Name | Description | License |
---|---|---|
Lodash | Provides utility functions for working with arrays, objects, strings | MIT |
React | Used for building user interfaces | MIT |
Express | Web application framework | MIT |
Axios | Promise-based HTTP client | MIT |
Adopting code reusability not only increases productivity but also enhances collaboration through shared resources among team members. It saves time by allowing developers to focus on unique aspects rather than rewriting repetitive codes repeatedly. By using standardized interfaces and modular designs, teams can easily integrate code snippets, libraries and open-source solutions.
Incorporating can help ensure that the software development process is efficient, cost-effective, and delivers quality results. The next step towards achieving software management system best practices is maintaining appropriate variable scope to prevent errors.
Maintaining appropriate variable scope to prevent errors
After implementing code reusability to reduce redundancy, the next crucial step is maintaining appropriate variable scope. This practice involves defining variables within a specific block of code where it is needed and avoiding global variables that can cause confusion and errors.
For example, consider an e-commerce website where customers can purchase items using their credit card information. If the developer uses global variables to store user data such as names and addresses, this could lead to security breaches and unauthorized access to sensitive information. By limiting the scope of these variables to only the necessary functions or classes, developers can prevent such risks.
Maintaining appropriate variable scope also helps in improving code readability and reducing maintenance costs. When all variables are defined within their respective functions or classes, it becomes easier for other developers to understand the purpose of each variable. Moreover, changes made in one part of the program do not affect other parts since they have limited access.
To achieve proper variable scoping, here are some best practices:
- Define variables in the smallest possible scope
- Avoid global variables unless absolutely necessary
- Use naming conventions that reflect a variable’s purpose
- Document your code clearly
Implementing these practices ensures consistency across different modules of your software system while promoting maintainability and scalability.
Pros | Cons |
---|---|
Easier debugging process | Increased memory usage |
Improved performance due to efficient use of resources | Longer development time |
Better team collaboration | Requires more planning upfront |
Adhering strictly to appropriate variable scoping standards may seem tedious at first but will ultimately save you time and effort in the long run. With , companies now realize how important proper coding standards are and how they impact overall productivity.
Organizing functions based on their purpose and functionality comes next after ensuring proper variable scoping techniques.
Organizing functions based on their purpose and functionality
As mentioned in the previous section, maintaining appropriate variable scope is crucial for software development. Another best practice that can enhance code quality is organizing functions based on their purpose and functionality.
For example, consider a software management system designed to track project timelines, budgets, and team member tasks. To ensure efficient and effective coding practices, it would be wise to group all time-related functions together, such as those responsible for calculating deadlines or tracking hours worked. Similarly, budget-related functions should also be grouped together so they are easier to locate and modify if necessary.
Organizing functions based on their purpose helps developers maintain modular code that is easy to read and understand. It also reduces clutter within files, which can make debugging much more manageable.
To further improve organization efforts, adopting consistent naming conventions across projects and teams has proven helpful in promoting clear communication among developers. This includes using descriptive function names that accurately reflect what each function does without being too lengthy or confusing. Additionally, variable names should clearly indicate what data they represent.
However, despite the benefits of organized code practices, some developers may resist implementing them due to perceived limitations on creativity or flexibility. To combat these concerns effectively while still ensuring the success of coding standards initiatives, managers must emphasize how organizational structures ultimately support innovation by making it easier for multiple individuals to work collaboratively and build upon existing code foundations.
In summary,:
- Organizing functions based on their purpose enhances modularity
- Consistent naming conventions promote clearer communication
- Some developers may resist organizational changes out of fear of losing creativity
- Managers must emphasize how structure supports collaboration
Next,{transition sentence into next section about Using descriptive function}.
Using descriptive function and variable names for clarity
Having organized functions based on their purpose and functionality, the next step is to use descriptive function and variable names for clarity. This ensures that anyone who reads your code will understand what each function does without having to read through its implementation details.
For example, let’s say you are developing a software management system for a car rental company. Instead of naming a function foo()
or bar()
, it would be better to name it something like calculateRentalFee()
or displayCustomerInformation()
. These names clearly convey the purpose of the function and make it easier for others to understand your code.
Using descriptive names not only helps other developers but also makes debugging easier. When an error occurs in your code, you can quickly identify which function caused the problem by looking at its name. This saves time and effort that would otherwise be spent searching through lines of code trying to find the issue.
To further improve readability and maintainability, here are some best practices when naming functions and variables:
- Use camelCase notation: The first word should be lowercase, while subsequent words should start with uppercase letters (e.g., calculateRentalFee).
- Be consistent with abbreviations: If you choose to abbreviate words in your names, make sure they are consistent throughout your codebase.
- Avoid using single-letter variable names: Unless they are used as loop counters or indices, single-letter variable names can be confusing.
- Use meaningful prefixes and suffixes: Adding prefixes such as “get” or “set” before function names adds context about their functionality.
In addition to naming conventions, adopting coding standards can have significant benefits for software development teams. Here are some ways that following coding standards can help improve team productivity:
Benefit | Description |
---|---|
Consistency | Following standardized guidelines ensures everyone writes similar-looking code that is easier for all members of the team to read and comprehend |
Code Quality | Consistent code is more likely to be of high quality and less prone to errors. |
Collaboration | Following guidelines can help team members collaborate better by reducing the time spent on reviewing each other’s work. |
Maintainability | Standardized code is easier to maintain, since everyone knows what to expect when making changes or adding new features. |
Adopting coding standards may seem like a tedious task initially, but it pays off in the long run by improving the overall quality of your codebase. By following best practices for naming functions and variables, you create code that is easily understood and maintained.
Implementing error handling and debugging techniques…
Implementing error handling and debugging techniques
In the previous section, we discussed how using descriptive function and variable names can improve code clarity. Let’s now explore another important aspect of coding standards: implementing error handling and debugging techniques.
Imagine a scenario in which you are working on a software project with multiple developers. One developer forgets to handle an exceptional case that leads to unexpected behavior in the program. The error is not caught until later testing phases, causing delays and frustration for everyone involved. This situation could have been avoided if proper error handling techniques were implemented from the start.
To ensure efficient and effective error handling, it is essential to follow best practices such as:
- Clearly defining acceptable input values and potential errors
- Using try-catch blocks to catch exceptions
- Providing informative error messages that help users diagnose issues
- Logging errors to track down bugs
Following these guidelines will not only prevent unnecessary delays but also minimize risks associated with poor-quality software such as data loss or security breaches.
When developing software, time is always of the essence; however, rushing through development without considering potential errors may lead to more significant setbacks in the long run. A recent study found that 75% of developers spend at least one day per week troubleshooting production problems caused by poor error handling practices. Therefore, investing time into proper debugging techniques early on can save resources over time.
One way to ensure consistent use of appropriate debugging methods across your team is by creating a standardized process for identifying faults and resolving them efficiently. Having clear procedures documented makes it easier for new team members to get up-to-speed quickly while maintaining quality control throughout your organization.
By establishing clear guidelines for debugging and error-handling within your programming team, you’ll enhance productivity while minimizing risk factors associated with low-quality programs. Check out this table below outlining some common best practices:
Best Practices | Description | Benefits |
---|---|---|
Define Acceptable Inputs & Errors | Clearly define valid input values and potential errors. | Prevents unexpected behavior |
Use Try-Catch Blocks | Catch exceptions in your code to prevent crashes or errors from propagating. | Code is more robust |
Provide Informative Error Messages | Help users diagnose issues by providing actionable error messages. | Users can fix problems faster |
Log Errors for Debugging | Track down bugs and identify patterns through logging errors. | Easier debugging |
In this section, we explored the importance of implementing error handling and debugging techniques when developing software programs. By following best practices such as defining acceptable inputs, using try-catch blocks, providing informative error messages, and logging errors, you can minimize risk factors associated with poor-quality software while enhancing productivity across your team.
Next up: Following established design patterns and principles {transition}.
Following established design patterns and principles
After implementing error handling and debugging techniques, the next best practice in software management is to follow established design patterns and principles. For instance, let’s consider a hypothetical case where a development team has been tasked with building an e-commerce platform from scratch. The first step would be to identify what design patterns are appropriate for this project.
One of the most popular design patterns used today is Model-View-Controller (MVC), which helps separate concerns between data management, user interface, and application logic. By using MVC, developers can create scalable applications that are easy to maintain over time. Another important principle is Don’t Repeat Yourself (DRY), which encourages code reuse and reduces duplication while keeping the overall system modular.
However, it’s not enough just to know these concepts; it’s essential to apply them consistently throughout the entire development process. Here are some essential tips:
- Ensure all team members understand these concepts by providing training sessions or resources.
- Use coding standards tools like linters or static analyzers as part of your continuous integration pipeline to ensure adherence.
- Encourage peer reviews of code changes before merging into the main branch.
- Consider conducting regular code audits to assess compliance with these guidelines.
By following standard practices such as these, teams can reduce technical debt, improve productivity, and enhance quality assurance efforts.
To further illustrate how adhering to coding standards contributes positively towards successful software management systems implementation we present this table highlighting notable benefits:
Benefit | Description |
---|---|
Improved Consistency | When everyone follows a specific set of rules when developing software components consistency will result in more readable cohesive codebases that are easier to maintain |
Better Collaboration | With consistent coding styles across multiple contributors working on shared projects collaboration becomes smoother – making it easier for others who come later down-the-line who may also work on the same project |
Enhanced Quality Assurance Processes | Using pre-established conventions during testing phases results in more efficient and effective quality assurance processes |
Reduced Technical Debt | Without coding standards, it is easy to accumulate technical debt over time. By following best practices from the start, teams can avoid this issue entirely or at least keep it under control |
In conclusion, adhering to established design patterns and principles is crucial for successful software management systems implementation. Teams that consistently follow these guidelines will reap the benefits of improved consistency, better collaboration, enhanced quality assurance processes, and reduced technical debt .
Using version control to manage code changes is another key component of software management best practices.
Using version control to manage code changes
Following established design patterns and principles is crucial to ensure the maintainability and scalability of software applications. However, it is equally important to use version control systems (VCS) to manage code changes efficiently.
For instance, imagine a scenario where a team of developers are working on an application without using VCS. One developer makes some changes that cause unexpected errors in the system. The team has no way of undoing those changes or identifying which specific modification caused the issue. This situation can lead to significant delays and confusion.
Using VCS allows teams to track every change made in the codebase, enabling them to identify issues quickly and revert problematic modifications easily. Additionally, VCS provides other benefits:
- Collaboration: Multiple developers can work on different parts of the same codebase simultaneously.
- Accountability: Developers are accountable for their contributions as all changes are logged with detailed information such as who made what modifications when.
- Experimentation: Teams can create branches for experimentation without affecting the main codebase.
- Backup: Code repositories act as backups ensuring that previous versions are always available if needed.
To effectively utilize VCS, teams must follow best practices such as committing frequently with descriptive messages, avoiding long-lived branches, and performing regular merges into the main branch.
Furthermore, there are several popular VCS options available today like Git, Mercurial, SVN among others. Each has its advantages and disadvantages depending on project requirements.
Pros | Cons |
---|---|
Distributed | Steep learning curve |
Fast performance | Can be overwhelming |
Supports branching | Requires more disk space |
While version control is essential for managing code changes efficiently, it’s not enough by itself. Development teams need proper coding standards in place so that everyone follows consistent guidelines while contributing to a project.
Implementing proper testing procedures is critical because it helps detect issues before they cause problems in production. The next section will cover best practices for testing software applications.
Implementing proper testing procedures
After implementing version control to manage code changes, the next essential practice for software management systems is proper testing procedures. For instance, let’s consider a hypothetical scenario where a company develops an e-commerce platform that enables customers to browse and purchase products online. Suppose the system has a critical bug in its payment gateway module that goes unnoticed during development and deployment. In that case, it could potentially result in financial loss, damaged reputation, and legal liabilities.
To avoid such scenarios, companies must follow industry-standard testing procedures before deploying their software into production environments. Here are some best practices for implementing proper testing procedures:
- Define test cases: Create a set of test cases that cover all possible use-cases of your software application. This includes functional tests to ensure the system meets business requirements and non-functional tests like performance, security, usability, compatibility with different devices/browser versions/operating systems.
- Automate Testing: Automate as much of the testing process as possible using tools like Selenium or Appium to execute repetitive tasks quickly and accurately across multiple platforms and browsers.
- Test Early & Often: Test early in the development lifecycle by integrating automated testing into continuous integration pipelines so that any bugs can be caught immediately rather than later when they become more difficult and costly to fix.
- Get Input from End-users: Involve end-users in beta-testing phases or user acceptance testing (UAT) so you can gather feedback on how real users interact with your product.
The importance of proper testing cannot be overstated; however, it’s not enough just to conduct tests regularly. You need to analyze test results effectively to identify areas requiring improvement continuously. One way you can achieve this goal is by creating dashboards or reports showing key metrics related to quality assurance efforts.
For example, here is a table detailing some common QA metrics:
Metric | Description | Formula |
---|---|---|
Defect Density | The number of defects per line of code or requirements | Total Number of Defects / Code Size (in lines) or Requirements Count |
Test Coverage | The percentage of the system covered by automated tests | Covered System Components / Total System Components x 100% |
Mean Time To Detect (MTTD) | How long it takes to detect a bug from its introduction into the system. | Sum(Time taken to detect bugs)/Total Bugs Detected |
Mean Time To Resolve (MTTR) | How long it takes to resolve an issue and deploy a fix. | Sum(Time taken to resolve issues)/Total Issues Resolved |
By tracking these metrics, you can gain insights into how effectively your team is identifying and fixing software bugs. Continuously optimizing code for performance and efficiency is critical in today’s fast-paced development environment.
Continuously optimizing code for performance and efficiency
After implementing proper testing procedures, the next step to ensure top-notch software management is continuously optimizing code for performance and efficiency. For example, let’s say a company developed a web application that was performing poorly due to slow page load times. The development team identified the root cause of the problem as inefficient code and took steps to optimize it.
To effectively optimize code, developers must follow coding standards best practices which include:
- Consistent naming conventions: all functions, variables, classes should have meaningful names.
- Clear commenting style: comments should explain why certain lines of code were added or removed.
- Minimizing dependencies: reducing external libraries used in coding can increase speed and reliability.
- Regularly refactoring: reviewing and improving existing code regularly helps avoid technical debt.
Following these guidelines ensures maintainability while helping mitigate unforeseen issues that may arise from poor optimization.
Another key component of optimizing code is staying up-to-date on emerging technologies. Developers need to be informed about new frameworks or tools that could improve their workflow. However, this does not mean jumping onto every latest trend without evaluating its potential impact on current workflows.
The table below highlights some popular front-end frameworks alongside their pros and cons:
Framework | Pros | Cons |
---|---|---|
ReactJS | High performance; robust community support. | Steep learning curve; boilerplate-heavy setup process. |
Vue.js | Simple syntax; great documentation; scalable architecture. | Limited ecosystem compared with ReactJS or AngularJS . |
AngularJS | Two-way data binding; dependency injection makes unit testing easier. | Code structure can become convoluted over time ; long-term support unclear since version update |
By considering both internal coding standards and emerging tech trends when developing software systems, companies can better position themselves against competitors by creating optimal user experiences through more efficient and higher-performing applications.
In conclusion, optimizing software systems requires following established coding standard best practices, staying informed of the latest trends in technology and evaluating their potential impacts on existing workflows. By doing so, companies can create better user experiences that translate to long-term customer satisfaction and a stronger market position.